python中的matplotlib库
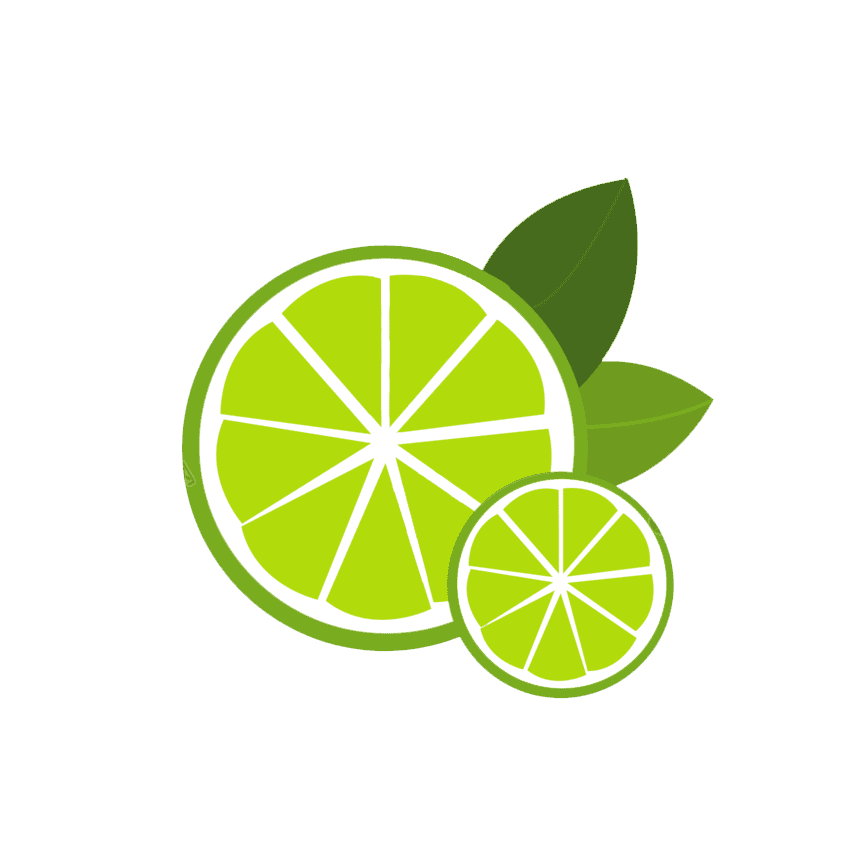
1. 核心功能
- 数据可视化:支持折线图、散点图、柱状图、饼图、3D 图等 20+ 种图表类型。
- 高度定制:可调整颜色、线型、标记、标签、标题、网格等样式。
- 跨平台输出:支持保存为 PNG、PDF、SVG 等格式,适配论文、网页、报告等场景。
2. 常用类与方法
pyplot
模块(类似 MATLAB 语法):1
2
3
4
5
6
7
8
9
10
11
12
13
14import matplotlib.pyplot as plt
# 绘制折线图
plt.plot([1, 2, 3, 4], [10, 20, 25, 30], 'r--o', label='Line 1')
# 设置标签、标题、图例
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.title("Simple Plot")
plt.legend()
# 显示网格和图表
plt.grid(True)
plt.show()Figure
和Axes
对象(面向对象风格):1
2
3
4
5
6
7
8
9
10
11fig = plt.figure(figsize=(8, 6)) # 创建画布
ax = fig.add_subplot(111) # 添加子图
ax.plot([1, 2, 3, 4], [10, 20, 25, 30], 'g:', label='Line 2')
ax.set_xlabel("X-axis")
ax.set_ylabel("Y-axis")
ax.set_title("Object-Oriented Plot")
ax.legend()
ax.grid(True)
plt.show()常用函数:
plt.scatter(x, y, s=None, c=None)
:绘制散点图,s
控制点大小,c
控制颜色。plt.bar(x, height, width=0.8)
:绘制柱状图。plt.pie(sizes, labels=None)
:绘制饼图。plt.hist(data, bins=10)
:绘制直方图。plt.savefig("plot.png", dpi=300, bbox_inches='tight')
:保存图表。
3. 使用示例
折线图:
1
2
3
4
5
6
7
8
9
10
11
12
13import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y, 'b-', linewidth=2, label='sin(x)')
plt.xlabel("X")
plt.ylabel("Y")
plt.title("Sine Wave")
plt.legend()
plt.grid(True)
plt.show()散点图:
1
2
3
4
5
6
7
8x = np.random.rand(50)
y = x + np.random.normal(0, 0.1, 50)
plt.scatter(x, y, s=50, c='red', edgecolor='black')
plt.xlabel("X")
plt.ylabel("Y")
plt.title("Scatter Plot")
plt.show()多子图:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20fig, axes = plt.subplots(2, 2, figsize=(10, 8))
# 子图 1
axes[0, 0].plot([1, 2, 3], [4, 5, 6], 'g--')
axes[0, 0].set_title("Subplot 1")
# 子图 2
axes[0, 1].scatter([1, 2, 3], [4, 5, 6], s=100, c='blue')
axes[0, 1].set_title("Subplot 2")
# 子图 3
axes[1, 0].bar(['A', 'B', 'C'], [10, 20, 30], color='lightgreen')
axes[1, 0].set_title("Subplot 3")
# 子图 4
axes[1, 1].hist(np.random.randn(1000), bins=30, color='purple')
axes[1, 1].set_title("Subplot 4")
plt.tight_layout()
plt.show()
4. 注意事项
- 安装问题:使用
pip install matplotlib
安装,若报错需检查依赖项(如 NumPy)。 - 中文显示:添加以下代码解决中文乱码:
1
2plt.rcParams['font.sans-serif'] = ['SimHei'] # 设置字体
plt.rcParams['axes.unicode_minus'] = False # 解决负号显示问题 - 图像保存:
- 使用
bbox_inches='tight'
避免图表被截断。 - 指定
dpi
提高分辨率(如dpi=300
)。
- 使用
- 性能优化:大数据量时避免频繁调用
plt.plot()
,改用plt.draw()
或分批绘制。
总结
- 基础绘图:用
plt.plot()
、plt.scatter()
、plt.bar()
等快速绘图。 - 样式调整:通过参数设置颜色、线型、标签等,或使用
plt.style.use('ggplot')
应用预定义样式。 - 多子图布局:用
plt.subplots()
创建复杂图表,plt.tight_layout()
自动调整间距。 - 输出图表:
plt.savefig()
保存为文件,plt.show()
直接显示。
- 标题: python中的matplotlib库
- 作者: lemon
- 创建于 : 2025-04-12 22:09:40
- 更新于 : 2025-04-12 22:13:21
- 链接: https://lemon2003.github.io/post/20250412220940.html
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。
评论