python中的tkinter库
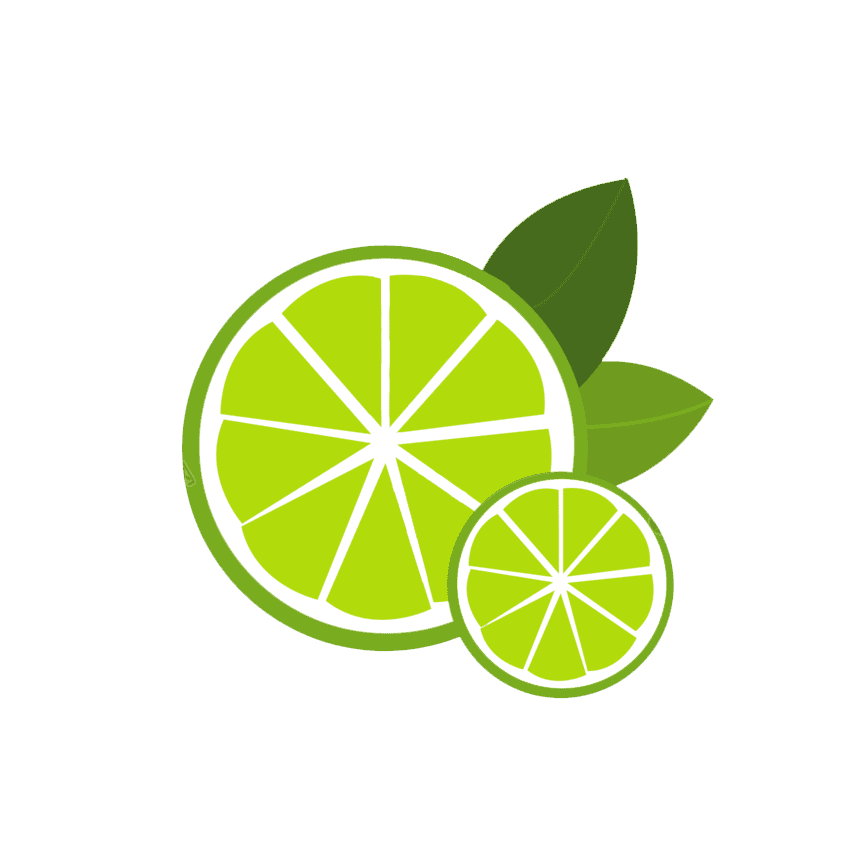
1. 核心功能
- GUI 开发:用于创建桌面应用程序的图形界面,支持按钮、标签、文本框等组件。
- 跨平台:可在 Windows、Linux、macOS 等系统上运行。
- 事件驱动:通过绑定事件(如点击、按键)实现用户交互。
2. 常用类与方法
主窗口类
Tk()
:root = tk.Tk()
:创建主窗口对象。root.title("标题")
:设置窗口标题。root.geometry("宽x高")
:设置窗口大小和位置。root.mainloop()
:启动主事件循环,保持窗口运行。
常用组件:
- 标签
Label
:1
2label = tk.Label(root, text="文本", font=("字体", 字号))
label.pack() # 添加到窗口 - 按钮
Button
:1
2
3
4def on_click():
print("按钮被点击")
button = tk.Button(root, text="点击", command=on_click)
button.pack() - 输入框
Entry
:1
2entry = tk.Entry(root)
entry.pack() - 文本框
Text
:1
2text = tk.Text(root)
text.pack() - 画布
Canvas
:1
2canvas = tk.Canvas(root, width=200, height=200)
canvas.pack()
- 标签
布局管理器:
pack()
:自动排列组件(简单布局)。grid()
:按网格行列布局(复杂布局)。place()
:绝对或相对坐标定位(精细控制)。
事件绑定:
1
2
3def on_key_press(event):
print("按下按键:", event.keysym)
root.bind("<Key>", on_key_press) # 绑定键盘事件
3. 使用示例
1 | import tkinter as tk |
4. 注意事项
- 主循环:必须调用
mainloop()
启动事件循环,否则窗口会立即关闭。 - 组件布局:组件需通过
pack()
、grid()
或place()
添加到窗口。 - 事件对象:事件处理函数需接收一个
event
对象,包含事件详细信息。 - 跨平台差异:某些功能(如窗口透明度、图标)在不同系统上表现可能不同。
总结
- 创建窗口:用
Tk()
初始化主窗口,设置标题和大小。 - 添加组件:使用
Label
、Button
、Entry
等类创建组件,并通过布局管理器添加到窗口。 - 处理事件:通过
bind()
方法绑定事件(如点击、按键)和处理函数。 - 启动循环:调用
mainloop()
保持窗口运行并处理用户交互。
- 标题: python中的tkinter库
- 作者: lemon
- 创建于 : 2025-04-12 22:03:14
- 更新于 : 2025-04-12 22:05:40
- 链接: https://lemon2003.github.io/post/20250412220314.html
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。
评论